什么是 Vue
Vue (发音为 /vjuː/,类似 view) 是一款用于构建用户界面的 JavaScript 框架。它基于标准 HTML、CSS 和 JavaScript 构建,并提供了一套声明式的、组件化的编程模型,帮助你高效地开发用户界面。
核心功能
- 声明式渲染:Vue 基于标准 HTML 拓展了一套模板语法,使得我们可以声明式地描述最终输出的 HTML 和 JavaScript 状态之间的关系。
- 响应性:Vue 会自动跟踪 JavaScript 状态并在其发生变化时响应式地更新 DOM。
声明式渲染:开发界面也是所见即所得
响应性: 比如最近关税问题,对不同国家的关税是有差异,应用就是当外部输入变化时能做出对应响应的东西
渐进式框架
Vue 是一个框架,也是一个生态。其功能覆盖了大部分前端开发常见的需求。但 Web 世界是十分多样化的,不同的开发者在 Web 上构建的东西可能在形式和规模上会有很大的不同。
目前想学习的是
- 单页应用 (SPA)
- 全栈 / 服务端渲染 (SSR)
API 风格
组合式
通过组合式 API,我们可以使用导入的 API 函数来描述组件逻辑。在单文件组件中,组合式 API 通常会与 <script setup>
搭配使用。这个 setup attribute 是一个标识,告诉 Vue 需要在编译时进行一些处理,让我们可以更简洁地使用组合式 API。
对于有面向对象语言背景的用户来说,这通常与基于类的心智模型更为一致
那选项式应该更适合我一点
选项式
使用选项式 API,我们可以用包含多个选项的对象来描述组件的逻辑,例如 data、methods 和 mounted。选项所定义的属性都会暴露在函数内部的 this 上,它会指向当前的组件实例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <script> export default { data() { return { count: 0 } },
methods: { increment() { this.count++ } },
mounted() { console.log(`The initial count is ${this.count}.`) } } </script>
<template> <button @click="increment">Count is: {{ count }}</button> </template>
|
如何创建一个Vue工程的应用
安装
前置一: Node.js
前提条件
熟悉命令行
已安装 18.3 或更高版本的 Node.js
终端输出Node.js
的版本
因此要先升级node
1 2 3 4 5
| $ brew list
... node ...
|
有node
,所以我原来是用brew
安装,也用brew
去升级
按照错误的解决方法来,有点慢本来想等等的,但是考虑以后,还是换下源
1 2 3
| https://mirrors.ustc.edu.cn/homebrew-core.git https://mirrors.aliyun.com/homebrew/homebrew-core.git https://mirrors.tuna.tsinghua.edu.cn/git/homebrew/homebrew-core.git
|
常用的几个源,ping了下值我这边最小的是aliyun的,环境问题只是准备工作,所以选以一个即可
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| $ cd "$(brew --repo)"/Library/Taps/homebrew/homebrew-cask
$ git remote set-url origin https://mirrors.ustc.edu.cn/homebrew-cask.git
$ git fetch --unshallow
$ cd "$(brew --repo)"/Library/Taps/homebrew/homebrew-core
$ git remote set-url origin https://mirrors.ustc.edu.cn/homebrew-core.git
$ git fetch --unshallow
|
brew update
完成后
更新node
看来升级有点问题,非关键问题,换种方式,搜了下也可以用n模块来安装
1 2 3 4 5 6 7
| $ sudo npm cache clean -f
$ sudo npm install -g n
$ sudo n stable
|
升级npm
1 2
| sudo npm install npm@latest -g
|
前置二: 开发工具 Visual Studio Code + Vue - Official 扩展
IDE按文档推荐的来
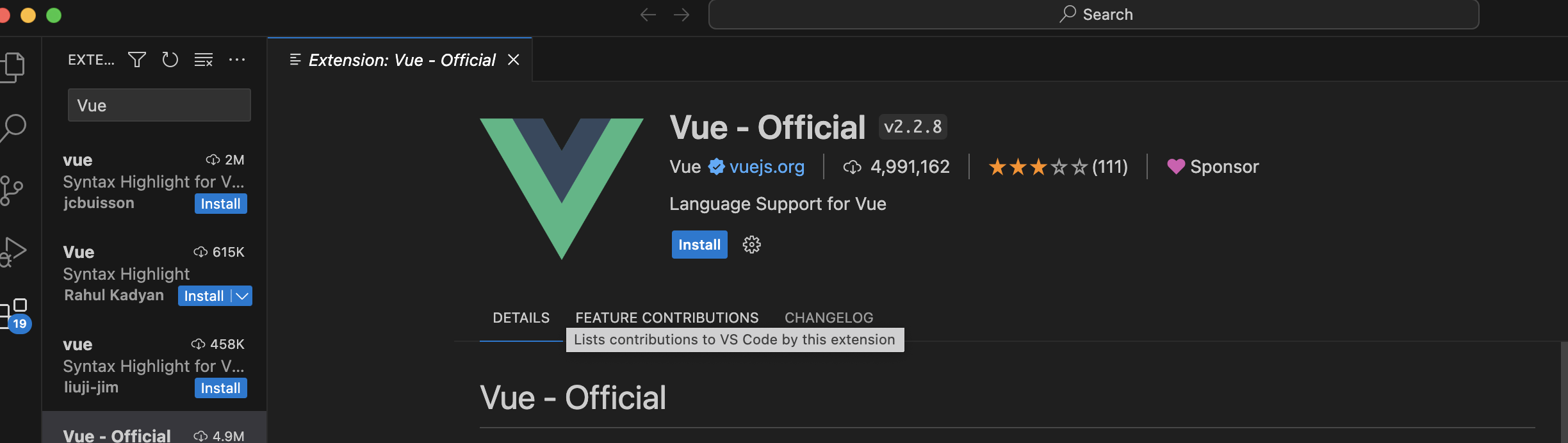
创建一个Vue应用
cd切到对应目录,然后在当前目录下创建一个Vue应用的
这一指令将会安装并执行 create-vue,它是 Vue 官方的项目脚手架工具。
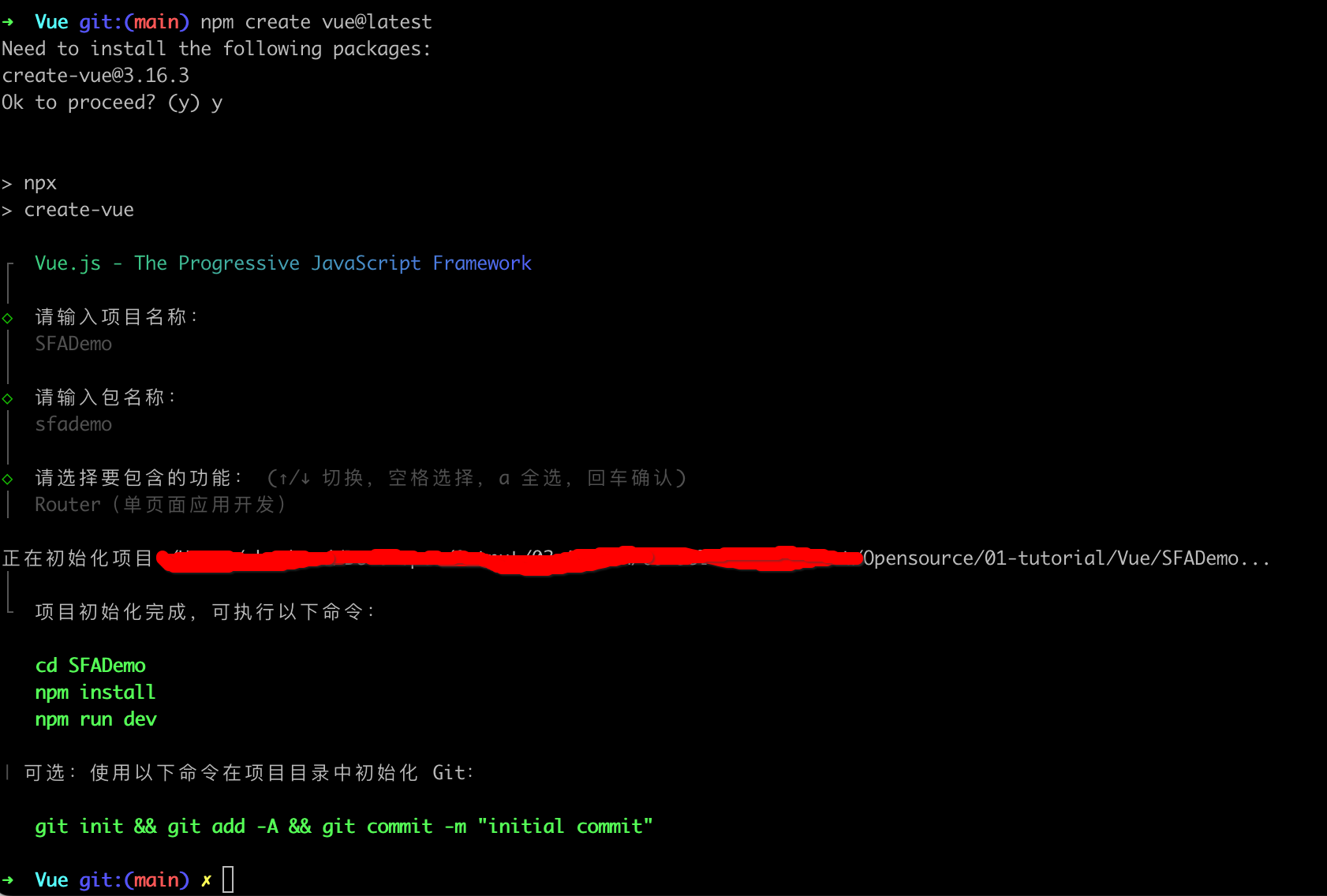
还有渐变色,有点好看😳
1 2 3 4 5 6
| cd SFADemo npm install npm run dev
|
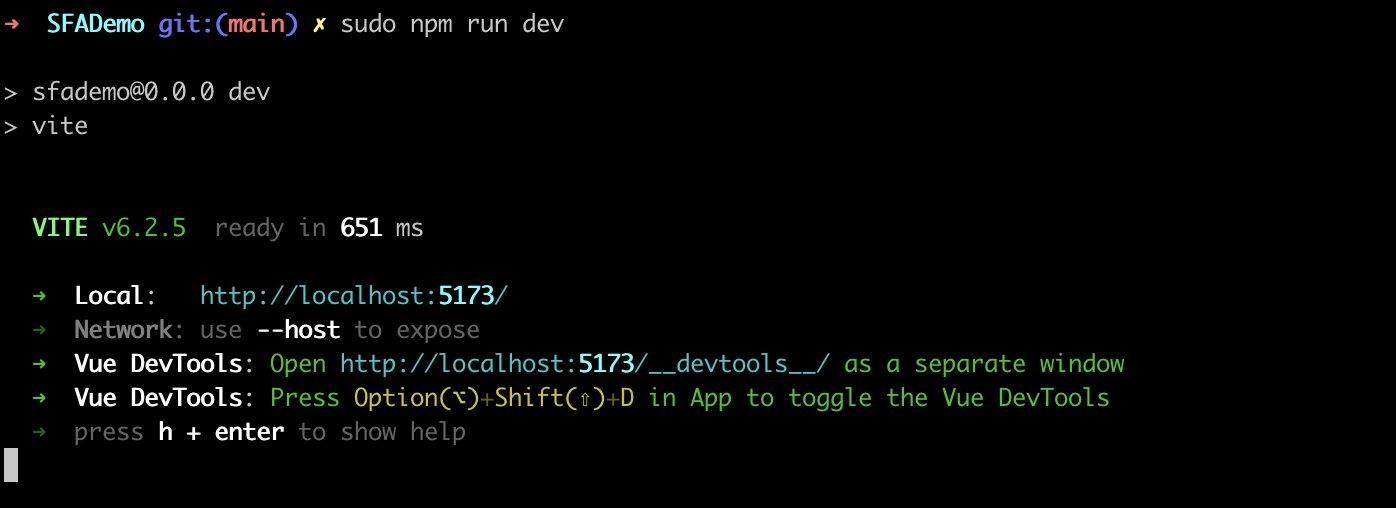
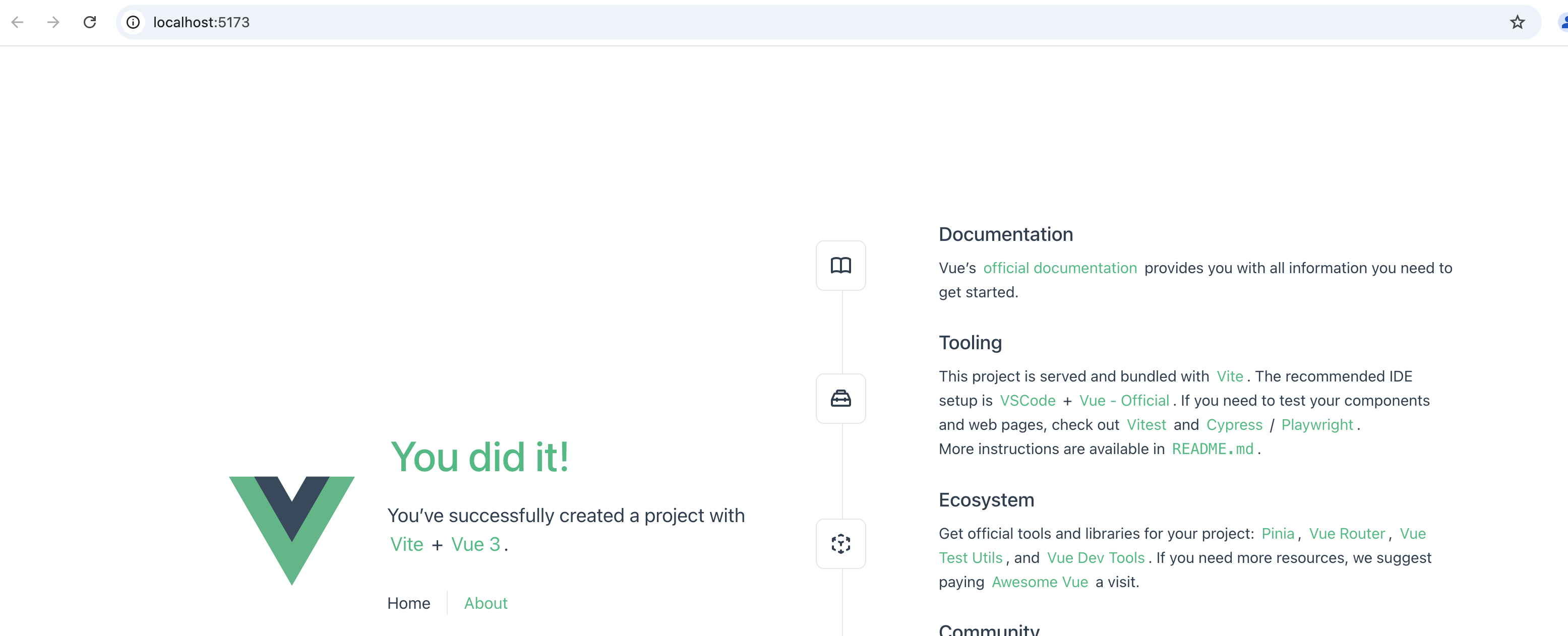
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13
| <!DOCTYPE html> <html lang=""> <head> <meta charset="UTF-8"> <link rel="icon" href="/favicon.ico"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vite App</title> </head> <body> <div id="app"></div> <script type="module" src="/src/main.js"></script> </body> </html>
|
好干净,Vite App
改成hello world
验证下
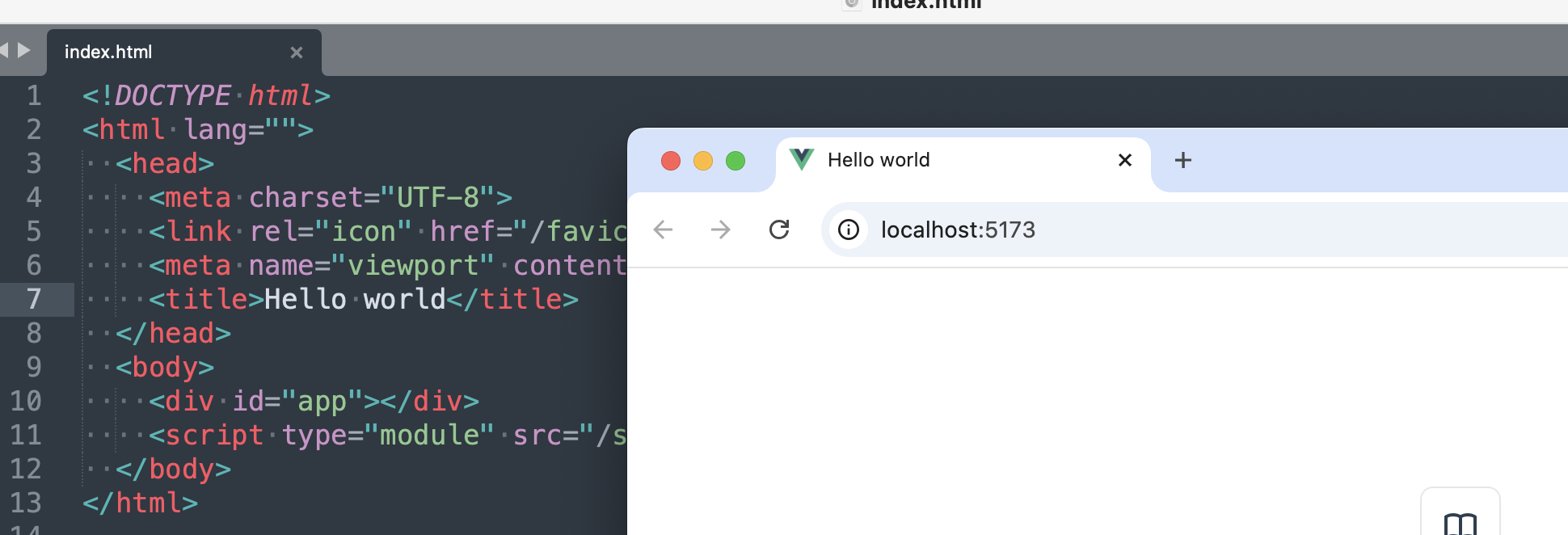
应用实例 + 根组件 + 挂载应用
入口是/src/main.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import './assets/main.css'
import { createApp } from 'vue' import App from './App.vue' import router from './router'
const app = createApp(App)
app.use(router)
app.mount('#app')
|
App.vue 里面是几组标签 javascript,style
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85
| <script setup> import { RouterLink, RouterView } from 'vue-router' import HelloWorld from './components/HelloWorld.vue' </script>
<template> <header> <img alt="Vue logo" class="logo" src="@/assets/logo.svg" width="125" height="125" />
<div class="wrapper"> <HelloWorld msg="You did it!" />
<nav> <RouterLink to="/">Home</RouterLink> <RouterLink to="/about">About</RouterLink> </nav> </div> </header>
<RouterView /> </template>
<style scoped> header { line-height: 1.5; max-height: 100vh; }
.logo { display: block; margin: 0 auto 2rem; }
nav { width: 100%; font-size: 12px; text-align: center; margin-top: 2rem; }
nav a.router-link-exact-active { color: var(--color-text); }
nav a.router-link-exact-active:hover { background-color: transparent; }
nav a { display: inline-block; padding: 0 1rem; border-left: 1px solid var(--color-border); }
nav a:first-of-type { border: 0; }
@media (min-width: 1024px) { header { display: flex; place-items: center; padding-right: calc(var(--section-gap) / 2); }
.logo { margin: 0 2rem 0 0; }
header .wrapper { display: flex; place-items: flex-start; flex-wrap: wrap; }
nav { text-align: left; margin-left: -1rem; font-size: 1rem;
padding: 1rem 0; margin-top: 1rem; } } </style>
|
import App from './App.vue'
为什么App是App? 改成Best
一样可以,那应该是当前场景下的约定。
先忽略怎么渲染的,大致是怎么显示出来?
1 2 3
| grep -r "official documentation" path/to/SFADemo
SFADemo/src/components/TheWelcome.vue:20: <a href="https://vuejs.org/" target="_blank" rel="noopener">official documentation</a>
|
所以怎么到TheWelcome.vue
1 2 3
| main.js App.vue -> HelloWorld.vue router/index.js -> HomeView.vue -> TheWelcomeItem.vue -> WelcomeItem.vue
|
参考
- 快速上手
- brew update 更新时报错
- Mac下安装,升级Node、npm
- 创建一个 Vue 应用